將 PDF 分割成多個(gè) PDF 文件
Spire.PDF for .NET 是一款專門對(duì) Word 文檔進(jìn)行操作的 .NET 類庫。致力于在于幫助開發(fā)人員輕松快捷高效地創(chuàng)建、編輯、轉(zhuǎn)換和打印 Microsoft Word 文檔,而無需安裝 Microsoft Word。
行號(hào)用于在每行文本旁邊顯示 Word 自動(dòng)計(jì)算的行數(shù)。當(dāng)我們需要參考合同或法律文件等文檔中的特定行時(shí),它非常有用。word中的行號(hào)功能允許我們?cè)O(shè)置起始值、編號(hào)間隔、與文本的距離以及行號(hào)的編號(hào)方式。使用 Spire.Doc,我們可以實(shí)現(xiàn)上述所有功能。本文將介紹如何將 HTML 轉(zhuǎn)換為 PDF。
歡迎加入spire技術(shù)交流群:767755948
在某些情況下,將單個(gè) PDF 文件分割成多個(gè)較小的 PDF 文件會(huì)很有幫助。例如,您可以將大型合同、報(bào)告、書籍、學(xué)術(shù)論文或其他文檔分割成小塊,以便于審查或重用。在本文中,您將學(xué)習(xí)如何使用 Spire.PDF for .NET 在 C# 和 VB.NET 中將 PDF 分割成單頁 PDF 以及如何按頁面范圍分割 PDF。
- 將 PDF 拆分為單頁 PDF
- 按頁面范圍分割 PDF
安裝 Spire.PDF for .NET
首先,您需要將 Spire.PDF for.NET 軟件包中包含的 DLL 文件作為引用添加到您的 .NET 項(xiàng)目中。這些 DLL 文件既可從此鏈接下載,也可通過 NuGet 安裝。
PM> Install-Package Spire.PDF
在 C#、VB.NET 中將 PDF 分割為單頁 PDF
Spire.PDF 提供的 Split() 方法可將多頁 PDF 文檔分割成多個(gè)單頁文件。以下是詳細(xì)步驟。
- 創(chuàng)建一個(gè) PdfDcoument 對(duì)象。
- 使用 PdfDocument.LoadFromFile() 方法加載 PDF 文檔。
- 使用 PdfDocument.Split(string destFilePattern, int startNumber) 方法將文檔分割為單頁 PDF 文件。
using System; using Spire.Pdf; namespace SplitPDFIntoIndividualPages { class Program { static void Main(string[] args) { //Specify the input file path String inputFile = "C:\\Users\\Administrator\\Desktop\\Terms of Service.pdf"; //Specify the output directory String outputDirectory = "C:\\Users\\Administrator\\Desktop\\Output\\"; //Create a PdfDocument object PdfDocument doc = new PdfDocument(); //Load a PDF file doc.LoadFromFile(inputFile); //Split the PDF to one-page PDFs doc.Split(outputDirectory + "output-{0}.pdf", 1); } } }[VB.NET]
Imports System Imports Spire.Pdf Namespace SplitPDFIntoIndividualPages Class Program Shared Sub Main(ByVal args() As String) 'Specify the path of the input file Dim inputFile As String = "C:\\Users\\Administrator\\Desktop\\Terms of Service.pdf" 'Specify the output directory Dim outputDirectory As String = "C:\\Users\\Administrator\\Desktop\\Output\\" 'Create a PdfDocument object Dim doc As PdfDocument = New PdfDocument() 'Load a PDF file doc.LoadFromFile(inputFile) 'Split the PDF to one-page PDFs doc.Split(outputDirectory + "output-{0}.pdf", 1) End Sub End Class End Namespace
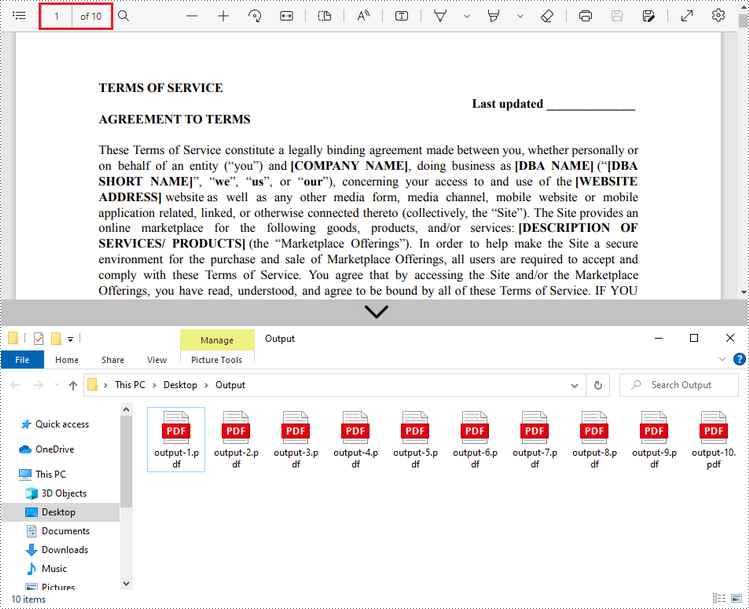
在 C#、VB.NET 中按頁面范圍分割 PDF
沒有提供按頁面范圍分割 PDF 文檔的直接方法。為此,我們需要?jiǎng)?chuàng)建兩個(gè)或多個(gè)新的 PDF 文檔,并將源文件中的頁面或頁面范圍導(dǎo)入其中。以下是詳細(xì)步驟。
- 加載源PDF文件,同時(shí)初始化PdfDocument對(duì)象。
- 創(chuàng)建兩個(gè)額外的PdfDocument對(duì)象。
- 使用PdfDocument.InsertPage()方法從源文件中導(dǎo)入第一頁到第一個(gè)文檔中。
- 使用 PdfDocument.InsertPageRange() 方法從源文件中導(dǎo)入其余頁面到第二個(gè)文檔中。
- 使用 PdfDocument.SaveToFile() 方法將兩個(gè)文檔分別保存為 PDF 文件。
[C#]
using Spire.Pdf; using System; namespace SplitPdfByPageRanges { class Program { static void Main(string[] args) { //Specify the input file path String inputFile = "C:\\Users\\Administrator\\Desktop\\Terms of Service.pdf"; //Specify the output directory String outputDirectory = "C:\\Users\\Administrator\\Desktop\\Output\\"; //Load the source PDF file while initialing the PdfDocument object PdfDocument sourceDoc = new PdfDocument(inputFile); //Create two additional PdfDocument objects PdfDocument newDoc_1 = new PdfDocument(); PdfDocument newDoc_2 = new PdfDocument(); //Insert the first page of source file to the first document newDoc_1.InsertPage(sourceDoc, 0); //Insert the rest pages of source file to the second document newDoc_2.InsertPageRange(sourceDoc, 1, sourceDoc.Pages.Count - 1); //Save the two documents as PDF files newDoc_1.SaveToFile(outputDirectory + "output-1.pdf"); newDoc_2.SaveToFile(outputDirectory + "output-2.pdf"); } } }[VB.NET]
Imports Spire.Pdf Imports System Namespace SplitPdfByPageRanges Class Program Shared Sub Main(ByVal args() As String) 'Specify the input file path Dim inputFile As String = "C:\\Users\\Administrator\\Desktop\\Terms of Service.pdf" 'Specify the output directory Dim outputDirectory As String = "C:\\Users\\Administrator\\Desktop\\Output\\" 'Load the source PDF file while initialing the PdfDocument object Dim sourceDoc As PdfDocument = New PdfDocument(inputFile) 'Create two additional PdfDocument objects Dim NewDoc_1 As PdfDocument = New PdfDocument() Dim NewDoc_2 As PdfDocument = New PdfDocument() 'Insert the first page of source file to the first document NewDoc_1.InsertPage(sourceDoc, 0) 'Insert the rest pages of source file to the second document NewDoc_2.InsertPageRange(sourceDoc, 1, sourceDoc.Pages.Count - 1) 'Save the two documents as PDF files NewDoc_1.SaveToFile(outputDirectory + "output-1.pdf") NewDoc_2.SaveToFile(outputDirectory + "output-2.pdf") End Sub End Class End Namespace
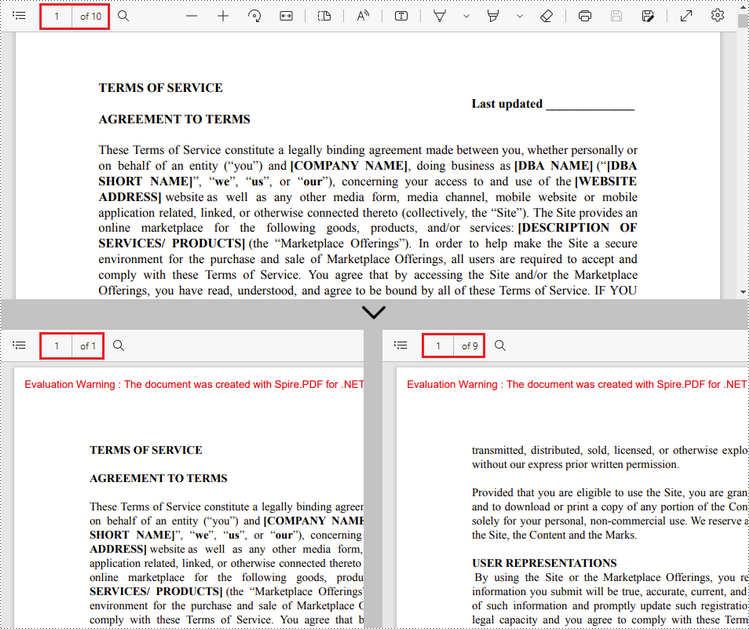
申請(qǐng)臨時(shí)許可證
若想從生成的文檔中刪除評(píng)估信息,或解除功能限制,申請(qǐng) 30 天試用許可證。